Next.js vs Traditional React: A Comprehensive Comparison for Web Developers
Discover the key differences between Next.js and traditional React in this comprehensive guide. Learn about rendering methods, SEO capabilities, routing, performance optimization, and use cases to choose the best framework for your project. Perfect for developers seeking clarity and insights!
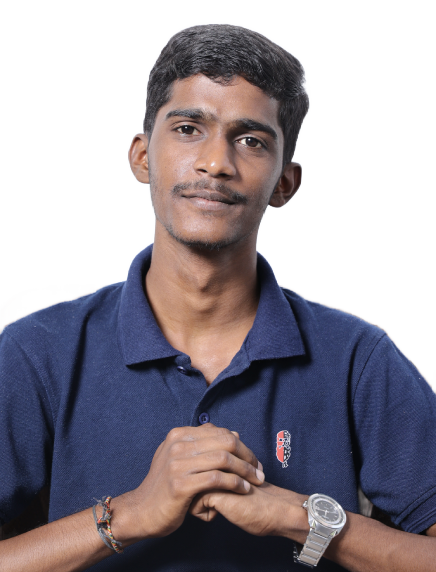
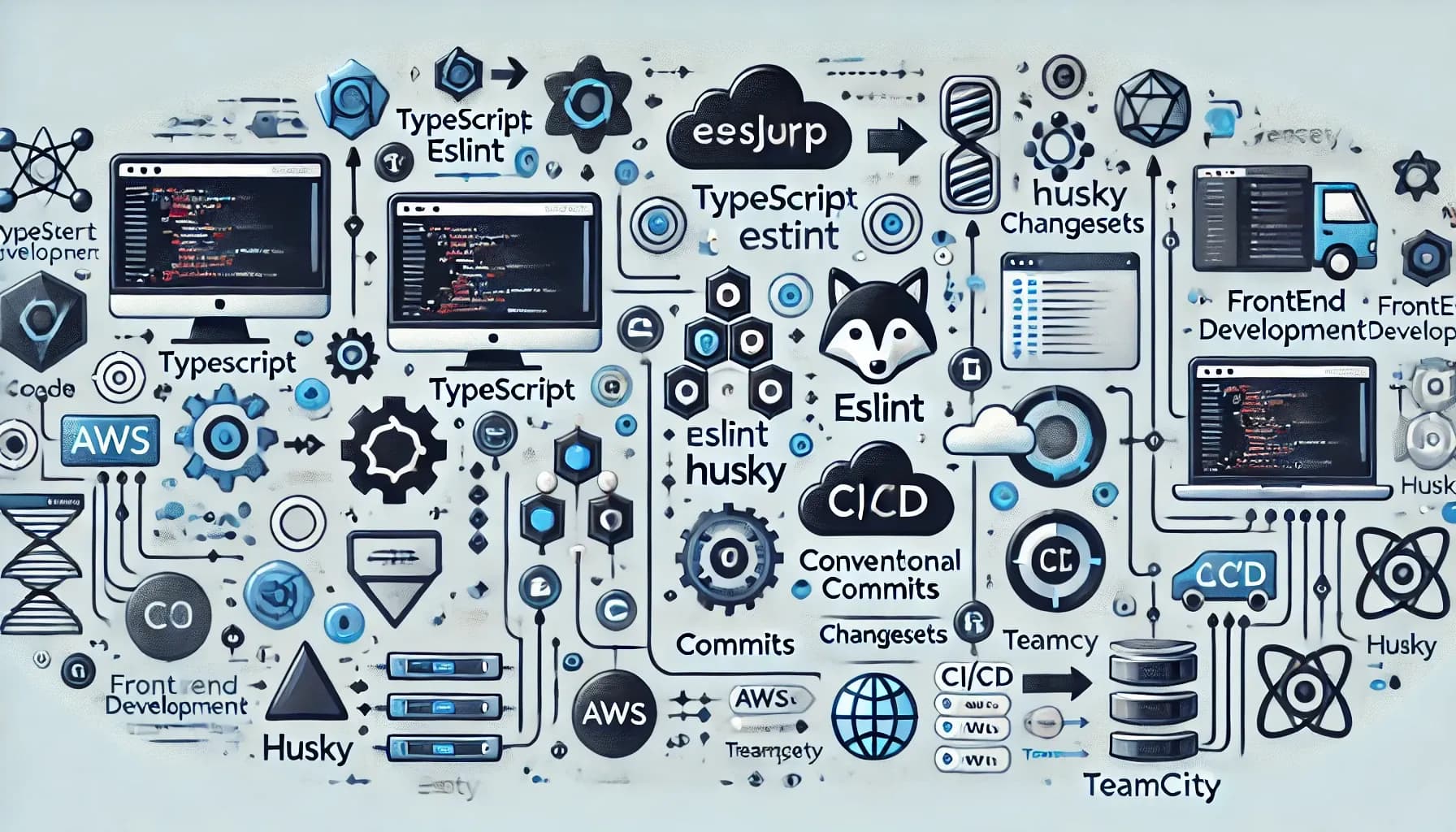
Introduction
In the rapidly evolving world of web development, choosing the right tools and frameworks is crucial for building efficient, scalable, and maintainable applications. React has been a game-changer since its inception, offering a powerful way to build user interfaces with its component-based architecture. However, as the demands for performance, SEO, and developer productivity increase, frameworks like Next.js have emerged to extend React’s capabilities.
This comprehensive article explores the intricacies of Next.js and traditional React, providing an extensive comparison to help you make informed decisions for your web development projects. We’ll dive deep into their architectures, features, performance considerations, development workflows, and more.
This comprehensive article explores the intricacies of Next.js and traditional React, providing an extensive comparison to help you make informed decisions for your web development projects. We’ll dive deep into their architectures, features, performance considerations, development workflows, and more.
or
Table of Contents
-
Understanding React and Next.js
- What is React?
- React’s Core Principles
- What is Next.js?
- Next.js Core Features
-
Detailed Feature Comparison
- Rendering Techniques
- Client-Side Rendering (CSR)
- Server-Side Rendering (SSR)
- Static Site Generation (SSG)
- Incremental Static Regeneration (ISR)
- Routing Mechanisms
- React Router
- Next.js File-System Routing
- Performance Optimization
- Code Splitting
- Lazy Loading
- Image Optimization
- Development Experience
- Tooling and Configuration
- Hot Module Replacement (HMR) and Fast Refresh
- TypeScript Support
- SEO and Metadata Management
- Meta Tags and Open Graph
- Sitemap and Robots.txt
- API Handling
- External APIs in React
- Next.js API Routes
- Internationalization (i18n)
- Testing and Debugging
- Deployment Strategies
- Rendering Techniques
-
In-Depth Pros and Cons
- Traditional React
- Next.js
-
Use Cases and Scenarios
- When to Choose Traditional React
- When to Choose Next.js
-
Migration Strategies
- Assessing Your Current React App
- Step-by-Step Migration Guide
-
Performance Benchmarks
- Load Times
- SEO Metrics
- User Experience
-
Case Studies and Real-World Examples
- Company X: Scaling with Next.js
- Startup Y: Flexibility with Traditional React
-
Future Developments
- React’s Roadmap
- Next.js Innovations
-
Conclusion
or
1. Understanding React and Next.js
What is React?
React is an open-source JavaScript library developed by Facebook and released in 2013. It focuses on building user interfaces (UIs) and is widely used for developing single-page applications (SPAs). React allows developers to create reusable UI components, manage state efficiently, and build complex interfaces with relative ease.
React’s Core Principles
• Declarative Programming: React promotes a declarative style of programming, where developers describe what the UI should look like rather than how to achieve it.
• Component-Based Architecture: The UI is broken down into small, reusable components that manage their own state and logic.
• Unidirectional Data Flow: Data flows in a single direction, making the application more predictable and easier to debug.
• Virtual DOM: React uses a virtual representation of the DOM to optimize updates and rendering, improving performance.
Example of a React Component:
jsx
import React from 'react'; function Greeting(props) { return <h1>Hello, {props.name}!</h1>; } export default Greeting;
What is Next.js?
Next.js is an open-source React framework created by Vercel (formerly Zeit) in 2016. It extends React by providing a robust set of features for building server-rendered and statically generated applications. Next.js aims to simplify the development process by offering built-in solutions for common challenges in React development.
Next.js Core Features
• Hybrid Rendering: Supports SSR, SSG, CSR, and ISR, allowing developers to choose the best rendering method for each page.
• File-System Routing: Simplifies routing by using the file system to generate routes automatically.
• API Routes: Enables the creation of backend endpoints within the Next.js application.
• Built-in CSS and Sass Support: Allows importing CSS files and using CSS Modules without additional configuration.
• Image Optimization: Provides automatic image optimization for improved performance.
• Internationalization (i18n): Offers built-in support for multiple languages and locales.
Example of a Next.js Page Component:
jsx
import React from 'react'; function Greeting(props) { return <h1>Hello, {props.name}!</h1>; } export default Greeting;
or
2. Detailed Feature Comparison
Rendering Techniques
Client-Side Rendering (CSR)
Traditional React:
• In CSR, the server sends a minimal HTML file with a <div id="root"></div>.
• The React application is bundled into JavaScript files, which the browser downloads and executes.
• The content is rendered on the client side after JavaScript execution.
Advantages:
• Rich client-side interactions.
• Simplified server setup.
• Efficient for applications where SEO is not a concern.
Disadvantages:
• Slower initial load times due to large JavaScript bundles.
• Poor SEO performance because search engine crawlers may not execute JavaScript.
Example of CSR Entry Point:
html
<!DOCTYPE html> <html> <head> <title>React App</title> </head> <body> <div id="root"></div> <script src="bundle.js"></script> </body> </html>
Server-Side Rendering (SSR)
Next.js:
• SSR generates the HTML on the server for each request.
• The initial page load includes fully rendered HTML, improving load times and SEO.
• After hydration, the React application takes over on the client side.
• The initial page load includes fully rendered HTML, improving load times and SEO.
• After hydration, the React application takes over on the client side.
Advantages:
• Faster initial render perceived by the user.
• Improved SEO as crawlers can access pre-rendered content.
• Better performance on slow devices and networks.
• Improved SEO as crawlers can access pre-rendered content.
• Better performance on slow devices and networks.
Disadvantages:
• Increased server load and complexity.
• Potentially longer time to first byte (TTFB).
• Potentially longer time to first byte (TTFB).
Example of SSR in Next.js:
jsx
export async function getServerSideProps(context) { // Fetch data on each request const data = await fetchData(); return { props: { data } }; } function Page({ data }) { return <div>{data.content}</div>; } export default Page;
Static Site Generation (SSG)
Next.js:
• SSG pre-renders pages at build time.
• Generated HTML files can be served over a CDN.
• Ideal for content that doesn’t change frequently.
• Generated HTML files can be served over a CDN.
• Ideal for content that doesn’t change frequently.
Advantages:
• Extremely fast load times.
• Reduced server costs due to pre-rendered pages.
• Enhanced security as there’s no server-side code execution on request.
• Reduced server costs due to pre-rendered pages.
• Enhanced security as there’s no server-side code execution on request.
Disadvantages:
• Not suitable for highly dynamic content.
• Requires rebuilding the site to update content.
• Requires rebuilding the site to update content.
Example of SSG in Next.js:
jsx
export async function getStaticProps() { const data = await fetchData(); return { props: { data } }; } function Page({ data }) { return <div>{data.content}</div>; } export default Page;
Incremental Static Regeneration (ISR)
Next.js:
• ISR allows updating static pages after deployment.
• You can revalidate pages at a specified interval.
• You can revalidate pages at a specified interval.
Advantages:
• Combines benefits of SSG and SSR.
• Fresh content without full site rebuilds.
• Fresh content without full site rebuilds.
Disadvantages:
• Complexity in cache management.
• Potentially stale data within the revalidation window.
• Potentially stale data within the revalidation window.
Example of ISR in Next.js:
jsx
export async function getStaticProps() { const data = await fetchData(); return { props: { data }, revalidate: 60, // Revalidate every 60 seconds }; } function Page({ data }) { return <div>{data.content}</div>; } export default Page;
Routing Mechanisms
React Router
Traditional React:
• React Router is the de facto standard for routing in React applications.
• Provides a client-side routing solution with dynamic route parameters and nested routes.
• Provides a client-side routing solution with dynamic route parameters and nested routes.
Advantages:
• Highly flexible and customizable.
• Supports complex routing scenarios.
• Extensive community support and documentation.
• Supports complex routing scenarios.
• Extensive community support and documentation.
Disadvantages:
• Requires additional setup and configuration.
• No automatic code splitting; manual setup needed.
• No automatic code splitting; manual setup needed.
Example of React Router Configuration:
jsx
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom'; function App() { return ( <Router> <Switch> <Route path="/about" component={AboutPage} /> <Route path="/users/:id" component={UserPage} /> <Route path="/" component={HomePage} /> </Switch> </Router> ); }
Next.js File-System Routing
Next.js:
• Routes are automatically generated based on the file structure in the pages directory.
• Supports dynamic routes with parameters and nested directories.
Advantages:
• Zero configuration required.
• Simplifies the routing setup.
• Automatic code splitting by route.
Disadvantages:
• Less flexibility for highly customized routing.
• May require workarounds for complex route structures.
Example of Next.js File-System Routing:
pages/ ├─ index.js // Renders at '/' ├─ about.js // Renders at '/about' ├─ users/ ├─ [id].js // Renders at '/users/:id'
Performance Optimization
Code Splitting
Traditional React:
• Requires manual configuration with tools like Webpack or Parcel.
• Developers must specify split points to break the app into smaller chunks.
Next.js:
• Automatic code splitting based on pages.
• Each page only loads the code it needs.
Example of Dynamic Import in React:
jsx
// Traditional React import React, { lazy, Suspense } from 'react'; const LazyComponent = lazy(() => import('./LazyComponent')); function App() { return ( <Suspense fallback={<div>Loading...</div>}> <LazyComponent /> </Suspense> ); }
Lazy Loading
• Both React and Next.js support lazy loading components to improve performance.
• Next.js enhances this with automatic code splitting and prefetching.
Image Optimization
Next.js:
• Provides an Image component for automatic image optimization.
• Supports resizing, lazy loading, and modern image formats like WebP.
Example of Next.js Image Component:
jsx
import Image from 'next/image'; function Profile() { return ( <Image src="/profile.jpg" alt="Profile Picture" width={500} height={500} /> ); }
Development Experience
Tooling and Configuration
Traditional React:
• Requires setup with tools like Create React App (CRA) or custom configurations.
• CRA provides a good starting point but may need ejecting for advanced customization.
Next.js:
• Comes with sensible defaults out of the box.
• Zero configuration needed for most use cases.
• Supports custom Webpack and Babel configurations if necessary.
Hot Module Replacement (HMR) and Fast Refresh
• Both React and Next.js support Fast Refresh for instant feedback during development.
• Next.js integrates Fast Refresh seamlessly without additional setup.
TypeScript Support
• Traditional React: Requires manual configuration to set up TypeScript.
• Next.js: Built-in support for TypeScript; automatically recognizes tsconfig.json.
SEO and Metadata Management
Meta Tags and Open Graph
Traditional React:
• Requires third-party libraries like react-helmet to manage <head> tags.
• Meta tags are injected after JavaScript execution, which may not be optimal for SEO.
• Meta tags are injected after JavaScript execution, which may not be optimal for SEO.
Next.js:
• Provides a built-in Head component to manage meta tags.
• Since Next.js supports SSR and SSG, meta tags are included in the initial HTML.
• Since Next.js supports SSR and SSG, meta tags are included in the initial HTML.
Example of Meta Tags in Next.js:
jsx
import Head from 'next/head'; function SEOPage() { return ( <> <Head> <title>SEO Optimized Page</title> <meta name="description" content="This is an SEO optimized page." /> </Head> <div>Content of the page.</div> </> ); }
Sitemap and Robots.txt
• Traditional React: Requires manual setup to generate sitemaps and robots.txt.
• Next.js: Can generate sitemaps during the build process, especially when using SSG.
API Handling
External APIs in React
• In traditional React, fetching data is typically done on the client side using fetch or libraries like Axios.
• For server-side data fetching, you’d need to set up a backend server.
Next.js API Routes
• Allows creating API endpoints directly within the Next.js application.
• Useful for handling form submissions, authentication, or custom backend logic.
• Useful for handling form submissions, authentication, or custom backend logic.
Example of an API Route in Next.js:
jsx
// pages/api/hello.js export default function handler(req, res) { res.status(200).json({ message: 'Hello from Next.js API!' }); }
Internationalization (i18n)
• Traditional React: Relies on third-party libraries like react-intl or react-i18next.
• Next.js: Offers built-in internationalization support, simplifying locale detection and routing.
Example of i18n Configuration in Next.js:
js
// next.config.js module.exports = { i18n: { locales: ['en-US', 'fr', 'de'], defaultLocale: 'en-US', }, };
Testing and Debugging
• Traditional React: Testing is commonly done with Jest and React Testing Library.
• Next.js: Supports the same testing frameworks, but additional considerations may be needed for SSR and API routes.
Deployment Strategies
Traditional React
• Static Hosting: Applications can be built into static files and hosted on services like GitHub Pages or Netlify.
• Server-Side Rendering: Requires setting up a Node.js server to handle SSR, increasing complexity.
Next.js
• Versatile Deployment Options:
• Static Export: Use next export to generate static HTML files.
• Serverless Deployment: Platforms like Vercel and Netlify support Next.js out of the box.
• Serverless Deployment: Platforms like Vercel and Netlify support Next.js out of the box.
• Custom Server: For advanced scenarios, you can set up a custom server with Express or Koa.
or
3. In-Depth Pros and Cons
Traditional React
Pros:
• Flexibility: Full control over the application’s structure and configuration.
• Ecosystem Richness: Extensive libraries and tools for state management, routing, and more.
• Community Support: Large community with abundant resources and tutorials.
• Simplified Hosting: Easy to deploy as static files for SPAs.
• Ecosystem Richness: Extensive libraries and tools for state management, routing, and more.
• Community Support: Large community with abundant resources and tutorials.
• Simplified Hosting: Easy to deploy as static files for SPAs.
Cons:
• SEO Limitations: Client-side rendering is not ideal for SEO-critical applications.
• Performance Issues: Potentially slower initial load times due to large JS bundles.
• Manual Configuration: Requires setup for routing, SSR, and code splitting.
• Increased Complexity for SSR: Implementing SSR without a framework can be challenging.
• Performance Issues: Potentially slower initial load times due to large JS bundles.
• Manual Configuration: Requires setup for routing, SSR, and code splitting.
• Increased Complexity for SSR: Implementing SSR without a framework can be challenging.
Next.js
Pros:
• Performance Enhancements: Faster initial loads through SSR and SSG.
• SEO Benefits: Pre-rendered content improves search engine indexing.
• Developer Productivity: Quick setup with zero configuration for routing and code splitting.
• Full-Stack Capabilities: Built-in API routes enable backend functionality.
• Advanced Features: Supports ISR, image optimization, and internationalization.
• SEO Benefits: Pre-rendered content improves search engine indexing.
• Developer Productivity: Quick setup with zero configuration for routing and code splitting.
• Full-Stack Capabilities: Built-in API routes enable backend functionality.
• Advanced Features: Supports ISR, image optimization, and internationalization.
Cons:
• Learning Curve: Developers need to understand SSR, SSG, and Next.js conventions.
• Less Flexibility: Adheres to certain conventions which may limit customization.
• Complexity for Simple Apps: Overhead may not be justified for small projects.
• Dependency on Node.js: Requires a Node.js environment for SSR and API routes.
• Less Flexibility: Adheres to certain conventions which may limit customization.
• Complexity for Simple Apps: Overhead may not be justified for small projects.
• Dependency on Node.js: Requires a Node.js environment for SSR and API routes.
or
4. Use Cases and Scenarios
When to Choose Traditional React
1. Single-Page Applications (SPAs):
• Example: Internal tools, dashboards, or applications where SEO is not a priority.
• Reasoning: Client-side rendering is sufficient, and the simplicity of deployment is advantageous.
2. High Customization Needs:
• Example: Projects requiring specific build configurations or unconventional architectures.
• Reasoning: Offers the flexibility to set up the project exactly as needed.
3. Learning and Prototyping:
• Example: Beginners learning React or developers building quick prototypes.
• Reasoning: Fewer abstractions help in understanding the core concepts of React.
4. Existing Ecosystem Integration:
• Example: Integrating React into an existing application or tech stack.
• Reasoning: Easier to incrementally adopt without overhauling the architecture.
When to Choose Next.js
1. SEO-Critical Applications:
• Example: E-commerce sites, blogs, news portals.
• Reasoning: SSR and SSG improve SEO and increase organic traffic.
• Reasoning: SSR and SSG improve SEO and increase organic traffic.
2. Performance-Focused Apps:
• Example: Applications targeting users on slow networks or devices.
• Reasoning: Faster initial load times enhance user experience and engagement.
• Reasoning: Faster initial load times enhance user experience and engagement.
3. Rapid Development:
• Example: Startups needing to get to market quickly.
• Reasoning: Out-of-the-box features reduce setup time and boilerplate code.
4. Full-Stack Development:
• Example: Small teams building both frontend and backend.
• Reasoning: API routes eliminate the need for a separate backend server.
• Reasoning: API routes eliminate the need for a separate backend server.
5. Static Websites with Dynamic Content:
• Example: Marketing sites that require frequent updates.
• Reasoning: ISR allows content updates without redeploying the entire site.
• Reasoning: ISR allows content updates without redeploying the entire site.
6. Internationalized Applications:
• Example: Global platforms serving multiple locales.
• Reasoning: Built-in i18n support simplifies localization efforts.
• Reasoning: Built-in i18n support simplifies localization efforts.
or
5. Migration Strategies
Assessing Your Current React App
Before migrating, evaluate:
• Project Complexity: Is your app complex enough to benefit from Next.js features?
• SEO Requirements: Do you need improved SEO performance?
• Performance Issues: Are users experiencing slow load times?
• Development Bottlenecks: Would built-in features like routing and code splitting enhance productivity?
• SEO Requirements: Do you need improved SEO performance?
• Performance Issues: Are users experiencing slow load times?
• Development Bottlenecks: Would built-in features like routing and code splitting enhance productivity?
Step-by-Step Migration Guide
1. Set Up a New Next.js Project:
bash
npx create-next-app@latest
2. Copy Existing Components:
• Move your components to the components/ directory in the Next.js project.
• Ensure all imports are updated accordingly.
• Ensure all imports are updated accordingly.
3. Update Routing:
• Convert your React Router setup to Next.js file-based routing.
• Create pages in the pages/ directory corresponding to your routes.
• Create pages in the pages/ directory corresponding to your routes.
4. Handle Static Assets:
• Place images, fonts, and other assets in the public/ directory.
• Update paths in your code to reference /assets/your-file.ext.
• Update paths in your code to reference /assets/your-file.ext.
5. Adjust Data Fetching Logic:
• Move data fetching to getStaticProps, getServerSideProps, or getStaticPaths as appropriate.
• Remove client-side data fetching where SSR or SSG is more suitable.
• Remove client-side data fetching where SSR or SSG is more suitable.
6. Migrate Global Styles and CSS Modules:
• Import global styles in pages/_app.js.
• Use CSS Modules for component-level styles.
• Use CSS Modules for component-level styles.
7. Test Each Page:
• Verify that each page renders correctly.
• Check for any client-side errors or warnings.
• Check for any client-side errors or warnings.
8. Optimize Performance:
• Utilize the next/image component for images.
• Ensure code splitting is effective by analyzing bundle sizes.
• Ensure code splitting is effective by analyzing bundle sizes.
9. Set Up API Routes (If Applicable):
• Move any backend logic into pages/api/ as API routes.
• Update frontend code to call these new endpoints.
• Update frontend code to call these new endpoints.
10. Configure SEO Metadata:
• Use the Head component to add meta tags for each page.
• Ensure titles and descriptions are unique and descriptive.
• Ensure titles and descriptions are unique and descriptive.
11. Finalize and Deploy:
• Test the application thoroughly in different environments.
• Deploy to a platform that supports Next.js, such as Vercel or Netlify.
• Deploy to a platform that supports Next.js, such as Vercel or Netlify.
or
6. Performance Benchmarks
Load Times
• Traditional React CSR:
• Time to First Paint (TTFP): Higher due to JavaScript parsing.
• Time to Interactive (TTI): Delayed until JavaScript is fully loaded.
• Time to Interactive (TTI): Delayed until JavaScript is fully loaded.
• Next.js SSR/SSG:
• TTFP: Lower because the HTML is pre-rendered.
• TTI: Faster as critical resources are loaded efficiently.
• TTI: Faster as critical resources are loaded efficiently.
Tools for Measurement:
• Lighthouse: Provides performance scores and metrics.
• WebPageTest: Offers detailed load time analysis.
• WebPageTest: Offers detailed load time analysis.
SEO Metrics
• Traditional React:
• May suffer from lower crawlability.
• Meta tags may not be recognized by search engines.
• Meta tags may not be recognized by search engines.
• Next.js:
• Improved crawlability due to pre-rendered content.
• Better ranking potential with proper SEO optimization.
• Better ranking potential with proper SEO optimization.
User Experience
• Perceived Performance:
• Users perceive Next.js apps as faster due to quicker content display.
• Accessibility:
• Both frameworks support accessibility best practices, but SSR can enhance screen reader compatibility.
or
7. Case Studies and Real-World Examples
Company X: Scaling with Next.js
Background:
• Company X runs an e-commerce platform with thousands of products.
• Faced issues with slow load times and poor SEO rankings.
• Faced issues with slow load times and poor SEO rankings.
Solution:
• Migrated to Next.js to leverage SSR and SSG.
• Implemented dynamic routes for product pages.
• Used ISR to update product information without redeploying.
• Implemented dynamic routes for product pages.
• Used ISR to update product information without redeploying.
Results:
• Improved page load times by 40%.
• Organic traffic increased by 25%.
• Enhanced user engagement and conversion rates.
• Organic traffic increased by 25%.
• Enhanced user engagement and conversion rates.
Startup Y: Flexibility with Traditional React
Background:
• Startup Y developed a real-time collaboration tool.
• Required complex client-side interactions and custom configurations.
• Required complex client-side interactions and custom configurations.
Solution:
• Chose traditional React for maximum flexibility.
• Customized Webpack configurations for specific needs.
• Focused on optimizing client-side performance.
• Customized Webpack configurations for specific needs.
• Focused on optimizing client-side performance.
Results:
• Achieved a highly interactive and responsive application.
• Maintained full control over the development stack.
• Rapid iterations without the overhead of SSR complexities.
• Maintained full control over the development stack.
• Rapid iterations without the overhead of SSR complexities.
or
8. Future Developments
React’s Roadmap
• Concurrent Mode:
• Enables React to prepare multiple versions of the UI at the same time.
• Improves responsiveness by rendering components without blocking the main thread.
• Improves responsiveness by rendering components without blocking the main thread.
• Suspense for Data Fetching:
• Simplifies asynchronous data fetching.
• Provides a declarative approach to loading states.
• Provides a declarative approach to loading states.
• Server Components:
• Allows components to be rendered on the server and sent to the client.
• Reduces bundle sizes and improves performance.
• Reduces bundle sizes and improves performance.
Next.js Innovations
• Middleware:
• Introduces the ability to run code before a request is completed.
• Useful for authentication, A/B testing, and redirects.
• Useful for authentication, A/B testing, and redirects.
• Edge Functions:
• Run code at the edge of the network, closer to the user.
• Enhances performance and reduces latency.
• Enhances performance and reduces latency.
• Improved Image Optimization:
• Continual enhancements to the next/image component.
• Support for more image formats and optimization techniques.
• Continual enhancements to the next/image component.
• Support for more image formats and optimization techniques.
or
9. Conclusion
The choice between Next.js and traditional React hinges on your project’s specific requirements and goals. Traditional React offers unparalleled flexibility and is well-suited for applications where SEO and initial load times are not critical. It allows developers to build highly interactive SPAs with full control over the application’s architecture.
Next.js, on the other hand, extends React’s capabilities by providing built-in features that address common challenges such as SEO, performance optimization, and routing. It streamlines development by reducing boilerplate code and simplifying complex tasks.
Key Takeaways:
• Opt for Traditional React if:
• Your application is an internal tool or dashboard.
• You need maximum flexibility and control.
• SEO is not a primary concern.
• You’re building a highly dynamic SPA.
• You need maximum flexibility and control.
• SEO is not a primary concern.
• You’re building a highly dynamic SPA.
• Opt for Next.js if:
• SEO and performance are paramount.
• You want a framework that handles SSR/SSG out of the box.
• Rapid development and built-in features are desirable.
• You’re building a content-heavy or e-commerce site.
• You want a framework that handles SSR/SSG out of the box.
• Rapid development and built-in features are desirable.
• You’re building a content-heavy or e-commerce site.
Ultimately, both frameworks are powerful tools in a web developer’s arsenal. Understanding their strengths and limitations allows you to leverage them effectively and build applications that meet your users’ needs.
or
10. Additional Resources
• Official Documentation:
• React Documentation
• Next.js Documentation
• Tutorials and Guides:
• React Official Tutorial
• Next.js Learn Course
• Building a Next.js App
• React Documentation
• Next.js Documentation
• Tutorials and Guides:
• React Official Tutorial
• Next.js Learn Course
• Building a Next.js App
• Books and Courses:
• Fullstack React by Accomazzo, Murray, and Lerner
• Mastering Next.js by Packt Publishing
• Fullstack React by Accomazzo, Murray, and Lerner
• Mastering Next.js by Packt Publishing
• Blogs and Articles:
• Understanding React’s Future with Concurrent Mode
• Next.js 12: The Future of Web Development
• Understanding React’s Future with Concurrent Mode
• Next.js 12: The Future of Web Development
or
We hope this comprehensive comparison has provided valuable insights to aid in your decision-making process. Whether you choose Next.js or traditional React, both offer robust solutions for modern web development. Feel free to share your thoughts, experiences, or questions!
dede
dede