Why TSX is More Powerful than JSX for Modern React Development
As React development continues to evolve, more developers are choosing TypeScript with JSX (TSX) over plain JSX. While JSX provides a straightforward syntax for JavaScript components, TSX adds the strong typing and developer tools of TypeScript, leading to safer, more maintainable code. In this article, we’ll explore why TSX is a powerful enhancement over JSX and how it can improve your React development experience. 1. Strong Typing with TypeScript * Error Prevention: TypeScript’s type-check
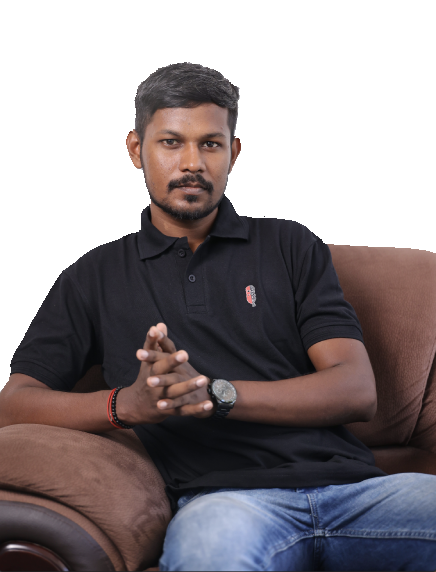
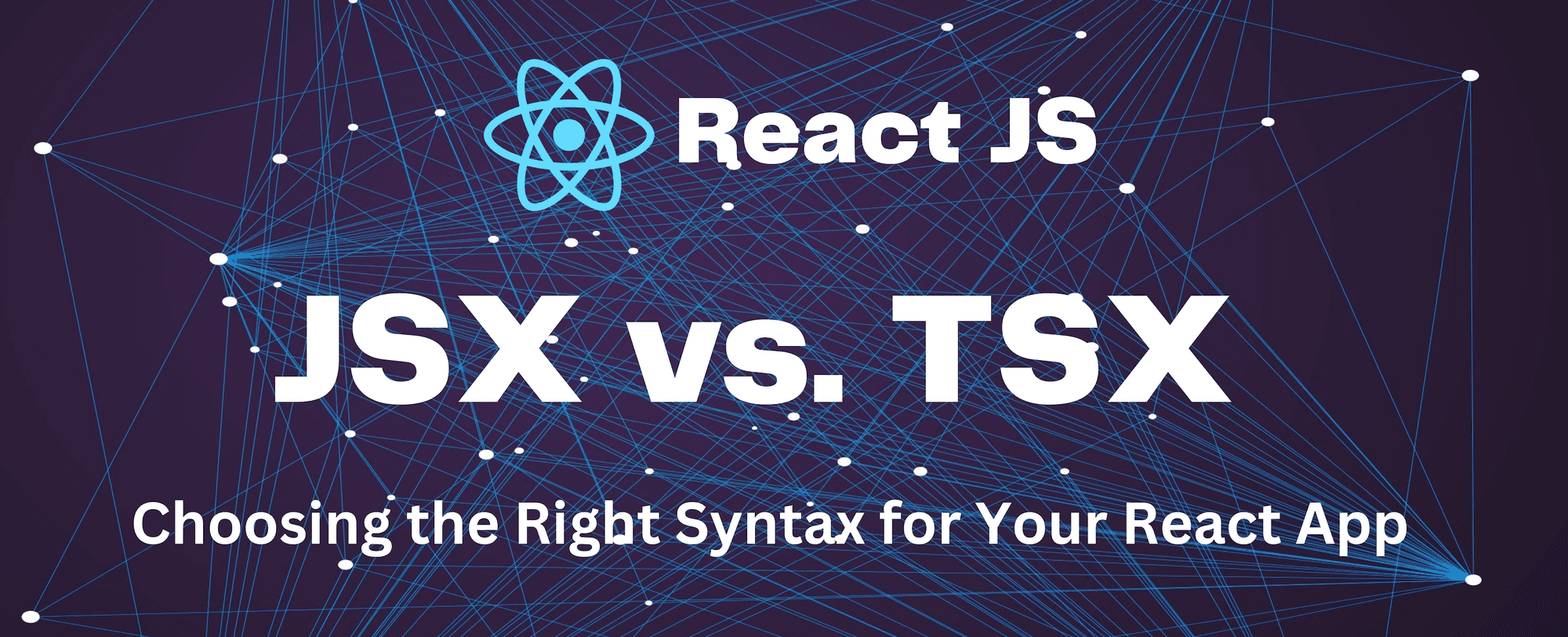
As React development continues to evolve, more developers are choosing TypeScript with JSX (TSX) over plain JSX. While JSX provides a straightforward syntax for JavaScript components, TSX adds the strong typing and developer tools of TypeScript, leading to safer, more maintainable code. In this article, we’ll explore why TSX is a powerful enhancement over JSX and how it can improve your React development experience.
1. Strong Typing with TypeScript
- Error Prevention: TypeScript’s type-checking can detect errors at compile time, which are usually found only during runtime with JSX. This prevents common bugs and improves code quality.
- Explicit Types: With TypeScript, you can define types for props, states, and other variables, ensuring you pass the correct data across components. TSX makes handling complex data models much safer and easier.
2. Enhanced Autocompletion and IntelliSense
- Better Developer Experience: With TSX, IDEs can provide richer autocompletion, code navigation, and error highlighting. This is especially useful in large projects where knowing function parameters, prop types, and component data shapes at a glance can speed up development.
- Fewer Documentation Checks: Type definitions in TSX reduce the need to constantly refer to documentation, allowing you to see the possible values and properties directly in your editor.
3. More Robust Component Prop Validation
- Avoids PropType Limitations: With TSX, you can enforce more complex prop structures than with React’s
PropTypes
. TypeScript lets you define union types, interfaces, and nested types, which are stricter and more versatile thanPropTypes
. - Compile-Time Checking: TSX offers compile-time prop validation rather than runtime, giving faster feedback when something goes wrong and preventing runtime errors in production.
4. Better Code Refactoring and Scalability
- Safer Refactoring: Strong typing makes it easier to refactor code because you can see where types or functions are used. TSX ensures that any changes to component props or state structures are reflected throughout the codebase, reducing manual errors during refactoring.
- Improved Scalability: As projects grow, maintaining loosely-typed code becomes challenging. TSX’s strict typing enforces consistent data structures and prop definitions, making it easier to manage and scale large applications.
5. Interfaces and Type Aliases for Props
- Reusability with Interfaces: TypeScript’s interfaces and type aliases allow you to define reusable data shapes, which can then be shared across multiple components. This leads to cleaner and more organized code.
- Composition Benefits: Using interfaces in TSX, you can compose and extend types across components, making data manipulation more consistent and allowing for more complex, flexible component hierarchies.
6. Better Handling of Asynchronous Code
- Promise Typing: With TypeScript, you can define exact types for asynchronous functions and promises, reducing errors when working with data fetching or other async operations.
- Error Handling: TypeScript’s type annotations help enforce error handling patterns in asynchronous code, ensuring that developers handle exceptions consistently.
7. Optional Chaining and Nullish Coalescing Support
- Null Safety: TypeScript offers optional chaining and nullish coalescing operators, which help prevent
null
andundefined
errors that are common in JavaScript. With TSX, you get these features alongside type-checking for improved code safety and reliability. - Type-Safe Conditionals: TSX lets you handle potential null values safely by ensuring conditions meet type requirements, reducing runtime exceptions and streamlining conditional rendering in JSX.
8. Code Consistency and Team Collaboration
- Unified Coding Standards: TypeScript enforces stricter coding standards, making it easier for teams to maintain code consistency. TSX’s type annotations ensure everyone adheres to the same data and structure standards.
- Easier Onboarding: When new developers join a project, TSX makes it easier to understand existing code with type hints, improving onboarding speed.
9. Error Boundaries and Better Error Management
- Type Safety in Error Boundaries: TSX allows you to use types for error boundaries, ensuring that error-handling code behaves as expected and handles component failures gracefully.
- Improved Debugging: TSX enables more precise error messages by ensuring type consistency, making it easier to trace issues back to the source and debug more effectively.
10. Type Inference and Code Optimization
- Automatic Type Inference: TypeScript can infer types based on context, reducing the need for explicit typing while still maintaining type safety.
- Code Optimization Opportunities: With TSX’s strict typing, optimizations can be done more effectively during transpilation, resulting in faster and more efficient code output.
or
Props & Cons using Tsx
Pros | Cons |
---|---|
Type Safety: Prevents errors by enforcing correct data types for props, reducing runtime bugs. | Increased Setup Complexity: Requires extra configuration, especially when adding TypeScript to an existing JavaScript codebase. |
Improved Developer Experience: Enhances autocompletion, IntelliSense, and inline documentation in IDEs, leading to faster development. | Steeper Learning Curve: TypeScript’s syntax and type annotations may be challenging for newcomers. |
Better Code Maintenance and Refactoring: Simplifies refactoring by ensuring prop changes are reflected consistently throughout the codebase. | Extra Development Time: Adding types to props can slow down initial development, especially for simple components. |
Enhanced Component Reusability: Enables reusable and consistent prop types with interfaces and type aliases across multiple components. | Verbose Syntax for Complex Types: Defining deeply nested or union types for props can lead to lengthy, complex code. |
Support for Complex Data Structures: Allows precise definition of complex data types, such as nested objects and union types, preventing misuse. | Potential Overhead for Small Projects: In small projects or simple components, TypeScript’s strict type-checking may feel like unnecessary overhead. |
or
Conclusion
While JSX provides an easy entry point to React development, TypeScript’s TSX adds layers of reliability, safety, and scalability that are essential for modern, complex applications. By integrating TypeScript, you not only minimize bugs but also create a more consistent and maintainable codebase that’s easier to collaborate on and scale. Adopting TSX can elevate your React development, future-proofing your projects and improving your overall development experience.