Understanding React Event Bubbling: Pros, Cons, and Key Facts
React event bubbling lets events propagate from child to parent, improving performance with one root-level listener. Benefits include efficient parent handling but can cause unintended triggers. stopPropagation and preventDefault help control bubbling effectively in React.
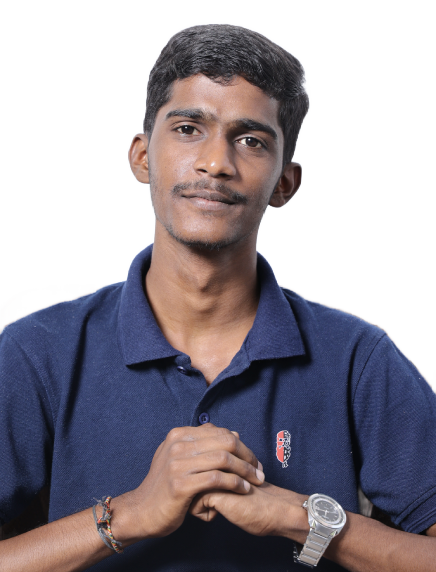
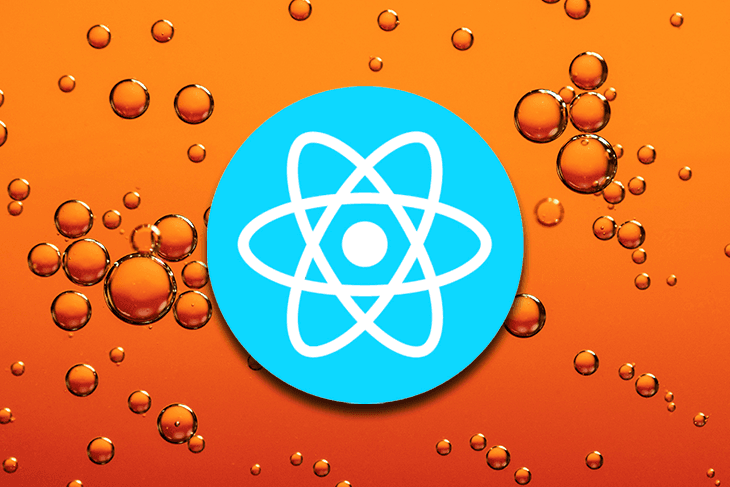
Event handling is an essential part of building interactive applications in React. One concept in event handling that developers encounter is event bubbling. In this article, we’ll dive deep into what event bubbling is, how it works in React, and its pros and cons. We’ll also discuss a few important and interesting facts that every React developer should know.
or
What is Event Bubbling?
Event bubbling is a concept in the DOM where an event starts from the target element and “bubbles” up to its ancestors in the DOM tree. For example, if you click a button inside a div, the click event on the button will also trigger on the div and continue to bubble up until it reaches the root of the DOM tree.
React uses synthetic events, which are cross-browser wrappers for native events, to handle events consistently across different browsers. Event bubbling in React works similarly to native DOM event bubbling but includes a few additional considerations due to React’s handling of the virtual DOM.
or
How Event Bubbling Works in React?
React uses a single event listener at the root of the document, known as delegated event handling. Instead of attaching individual listeners to each element, React attaches a single event listener to the root, such as document or root DOM node. When an event is triggered, React uses the virtual DOM to determine which component is responsible for the event.
This approach helps reduce memory usage and improve performance since only a single listener is needed to handle all events of a particular type. The synthetic event then bubbles up the component tree, allowing you to manage events effectively without attaching multiple listeners to each DOM element.
or
Pros of Event Bubbling in React
1. Performance Optimization: With React’s delegated event handling, there is only one event listener for each type of event (e.g., click, keydown). This reduces the number of event listeners attached to individual elements, enhancing performance and memory usage.
2. Easy Parent Event Handling: Event bubbling allows you to handle events at a parent level, capturing events triggered by child elements. This is useful for cases where you want to listen to multiple child elements with a single event handler.
3. Event Delegation: Event bubbling enables event delegation. You can attach a single event listener to a parent element that handles events for multiple child elements. This is particularly useful for handling dynamically added or removed elements without needing to add or remove event listeners individually.
4. Consistent Event API: React’s synthetic events provide a consistent API across browsers. This makes it easier for developers to handle events reliably across different environments.
or
Cons of Event Bubbling in React
1. Unexpected Event Triggers: Event bubbling can sometimes lead to unexpected event triggers, especially if multiple event handlers are attached to parent and child elements. For example, clicking a button inside a form might trigger the button’s click event as well as the form’s submit event unintentionally.
2. Overhead with Stop Propagation: To prevent an event from bubbling up, you need to explicitly call event.stopPropagation(). This can be tedious, especially when managing complex UI structures, as you need to keep track of where the propagation should and shouldn’t be stopped.
3. Event Overwriting: Sometimes, parent components might accidentally intercept or overwrite events that were intended for child components. This can create conflicts, especially in large applications where parent and child components handle similar events (like click).
4. Complexity with Nested Components: In React, components are often deeply nested. When using event bubbling with deeply nested components, tracking the event’s journey through the component tree can become complex and challenging to debug.
or
Practical Example of Event Bubbling in React
Here’s an example to illustrate event bubbling in React:
tsx
import React from 'react'; const App = () => { const handleParentClick = () => { alert("Parent div clicked!"); }; const handleChildClick = (event) => { event.stopPropagation(); // Prevents bubbling to the parent alert("Child div clicked!"); }; return ( <div onClick={handleParentClick} style={{ padding: "20px", border: "2px solid black" }}> <h2>Parent Div</h2> <div onClick={handleChildClick} style={{ padding: "20px", border: "2px solid red" }}> <p>Child Div</p> </div> </div> ); }; export default App;
In this example:
• Clicking on the child div will trigger handleChildClick, and the stopPropagation() method prevents the event from bubbling up to the parent div.
• Without stopPropagation(), clicking the child div would trigger both handleChildClick and handleParentClick.
or
Key Facts and Interesting Aspects
1. Synthetic Events: React’s synthetic events are designed to mimic the native events, and they use event pooling. This means that synthetic events are reused across multiple events for performance optimization. However, event pooling has been deprecated in React 17, so this is mostly relevant for earlier versions.
2. Event Delegation by Default: React’s delegated event handling (attaching one listener for each type of event) is one reason React applications often have better performance in event-heavy scenarios compared to direct DOM event listeners.
3. React 17 Changes: In React 17, event bubbling behaviour changed slightly to allow attaching native events outside the React root component without interfering with React’s synthetic event system. This change makes it easier to use React alongside other libraries that rely on native DOM events.
4. stopPropagation and preventDefault: Both of these methods can be used with React synthetic events. stopPropagation stops the event from bubbling up, while preventDefault prevents the default action (e.g., preventing form submission).
or
Pros and Cons Summary Table
Pros | Cons |
---|---|
Improves performance through event delegation | Can lead to unexpected event triggers |
Enables easy event handling for parent nodes | Requires stopPropagation for granular control |
Reduces memory usage | Event conflicts with parent and child handlers |
Consistent cross-browser event handling | Complex debugging with deeply nested components |
or
Final Thoughts
Event bubbling in React, managed through synthetic events, offers significant performance benefits and ease of handling. However, it requires careful handling to avoid unintended behaviour, especially when working with deeply nested components. By understanding how React’s event system works and when to use stopPropagation or preventDefault, you can take full advantage of event bubbling in your applications.
Whether you’re building a simple UI or a complex component tree, understanding event bubbling is key to effectively managing events in React.