Bridging the Gap Between Design and Code: How Storybook Supports Teams
In modern development workflows, collaboration between designers and developers has become crucial. However, ensuring smooth communication between these two disciplines is often a challenge. Designers craft beautiful interfaces, and developers transform those designs into functional code. But how can teams bridge the gap between design concepts and their code implementation without unnecessary confusion or delays? What is Storybook? Storybook is an open-source tool that allows you to develop,
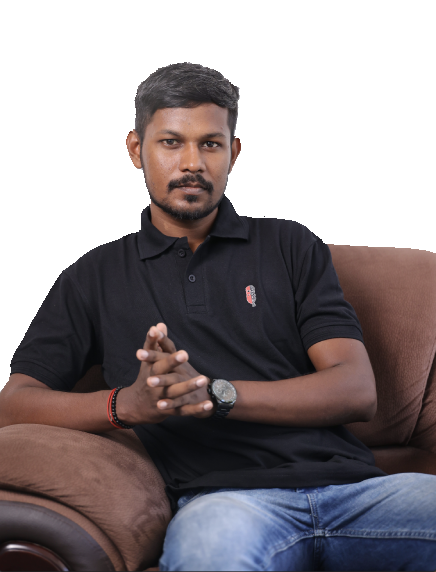
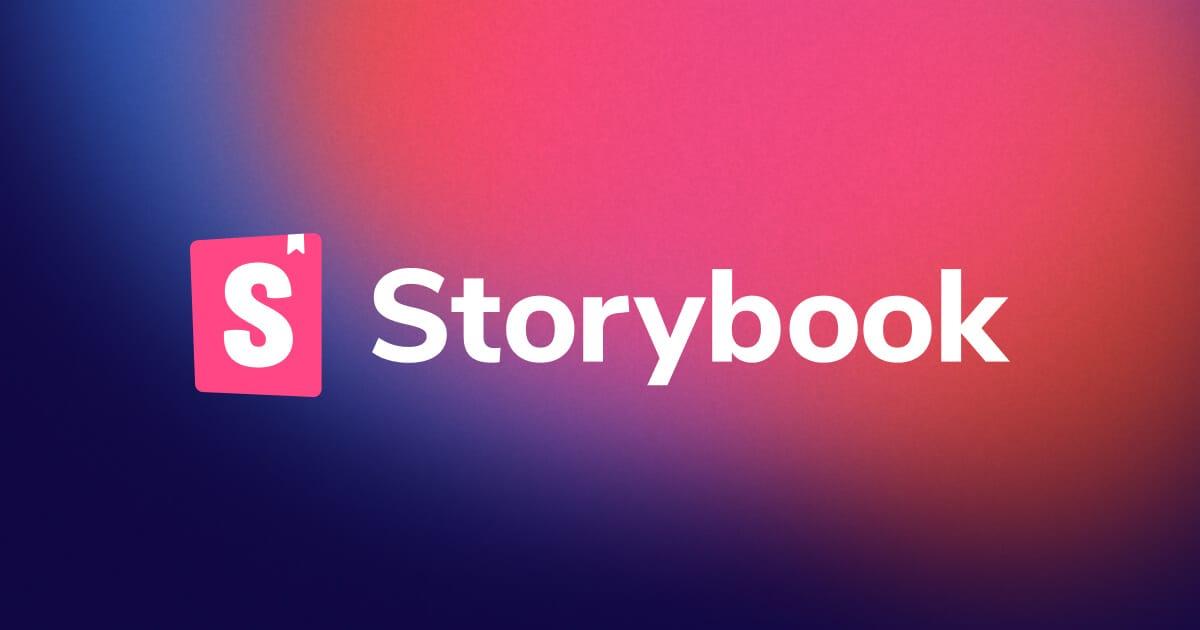
In modern development workflows, collaboration between designers and developers has become crucial. However, ensuring smooth communication between these two disciplines is often a challenge. Designers craft beautiful interfaces, and developers transform those designs into functional code. But how can teams bridge the gap between design concepts and their code implementation without unnecessary confusion or delays?
What is Storybook?
Storybook is an open-source tool that allows you to develop, test, and showcase UI components in isolation. It is especially popular in frontend development for building and maintaining UI components independently of the main application. Storybook provides a live, interactive interface where developers can view and interact with components in various states, making it a valuable tool for both individual developers and teams working on user interfaces.
Storybook helps in creating a component library where all the UI components are stored and documented. It supports multiple frameworks, such as React, Vue, Angular, Svelte, and others, making it versatile and suitable for various frontend projects
or
The Challenge of Collaboration
Traditionally, turning designs into code has been a back-and-forth process between designers and developers. Designers hand over their mockups, and developers try to interpret and implement them as accurately as possible. However, this often leads to misinterpretation, inconsistency, and tedious revisions.
Storybook helps solve these challenges by providing a shared platform where both designs and code exist side by side, enabling more seamless collaboration between teams.
Why Use Storybook?
- Component Isolation: Storybook allows you to develop and test UI components in isolation, which simplifies debugging and enhances focus on individual elements of the UI.
- Documentation: Storybook serves as living documentation for your UI components, showcasing their states and variations. This helps teams understand how each component should behave.
- Collaboration: It enables designers and developers to work with a shared understanding of the UI components. This reduces back-and-forth and ensures a more consistent UI/UX.
- Rapid Prototyping: Storybook allows quick testing of design ideas and prototypes without the need to set up a full app environment.
or
How to Integrate Storybook into Your Project
Here’s a step-by-step guide to integrating Storybook into a JavaScript or TypeScript project (with React as an example):
1️⃣ Install Storybook
First, install Storybook as a dependency in your project. Open your terminal and navigate to your project directory, then run the following command:
sh
npx storybook init
This command will automatically install Storybook and its dependencies in your project.
Alternatively, if you prefer to manually install Storybook, use these commands (assuming you’re using npm for package management):
sh
npm install @storybook/react --save-dev
If you are using Yarn:
sh
yarn add @storybook/react --dev
2️⃣ Configure Storybook
Once installed, you should see a
.storybook
folder and several configuration files created in your project directory, such as main.js
, preview.js
, and manager.js
. The default configuration should work well for most setups, but you can customize these files as needed.For example, in
main.js
, you can specify where to find your stories (UI components):module.exports = {
stories: ['../src/**/*.stories.js'],
addons: [
'@storybook/addon-links',
'@storybook/addon-essentials',
'@storybook/addon-interactions',
],
};
stories: ['../src/**/*.stories.js'],
addons: [
'@storybook/addon-links',
'@storybook/addon-essentials',
'@storybook/addon-interactions',
],
};
3️⃣ Create Stories for Your Components
Storybook works by creating stories for your components. A story is a single state or variation of a component, like a button with different text, size, or color.
In your component directory, create a
.stories.js
(or .stories.tsx
for TypeScript) file alongside the component file. Here's an example for a simple Button
component:Button.js
(your component):
html
import React from 'react'; const Button = ({ label, onClick }) => ( <button onClick={onClick}>{label}</button> ); export default Button;
Button.stories.js
(the Storybook story):
html
import React from 'react'; import Button from './Button'; // Default story for Button component export default { title: 'Button', component: Button, }; export const Default = () => <Button label="Click Me" />; export const Disabled = () => <Button label="Disabled" disabled />;
4️⃣ Run Storybook
Once your stories are created, you can run Storybook to view your components in isolation. From your project root directory, run:
sh
npm run storybook
This command will start Storybook in development mode, typically on
http://localhost:6006/
. Open this URL in your browser, and you'll be able to see your components rendered in the Storybook UI.5️⃣ Add More Stories and Component States
You can continue adding new stories to reflect different states, variations, or configurations of your components. This is useful for seeing how your component behaves under different conditions, such as with different props, inputs, or actions.
Here’s an extended example of a
Button.stories.js
:html
import React from 'react'; import Button from './Button'; export default { title: 'Button', component: Button, }; export const Default = () => <Button label="Click Me" />; export const Primary = () => <Button label="Primary Button" style={{ backgroundColor: 'blue' }} />; export const Disabled = () => <Button label="Disabled" disabled />; export const Large = () => <Button label="Large Button" style={{ padding: '20px 40px' }} />;
6️⃣ Deploy Storybook (Optional)
If you want to share your component library or showcase it, you can deploy Storybook. You can host it on platforms like GitHub Pages, Netlify, or Vercel.
For example, to deploy to GitHub Pages, you can use the following commands:
- Build Storybook for production:
sh
npm run build-storybook
- Deploy the generated static files to GitHub Pages using a tool like
gh-pages
:
sh
npm install --save-dev gh-pages
In your
package.json
, add a deploy script:json
"scripts": { "deploy": "gh-pages -d storybook-static" }
Then, run the deploy script:
sh
npm run deploy
or
Conclusion
Storybook is a powerful tool that simplifies the development and documentation of UI components. By integrating Storybook into your project, you can isolate components, improve collaboration between teams, and create a robust component library that can be reused across your projects. Whether you're building a small app or a large-scale enterprise system, Storybook enhances the development experience and ensures consistency across your UI.
If you need more guidance on specific frameworks or configurations, Storybook has extensive documentation to help you get the most out of it.